Call Azure API with Powershell
Table of content
If you want to create your own or to contribute to an existing GitHub project you are on the right page.
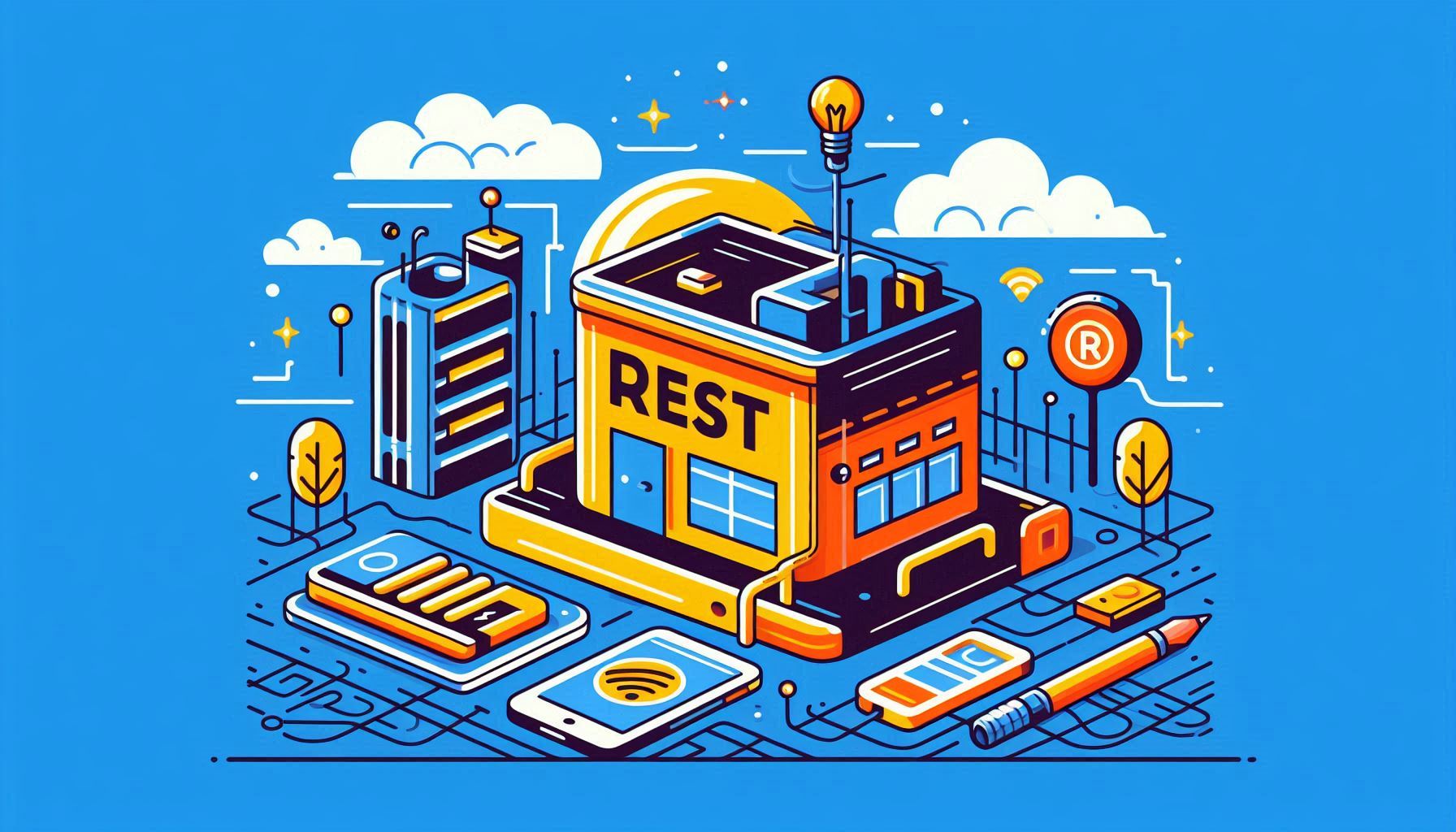
Using PowerShell or the command line to call an Azure REST API is a quick method to retrieve or update information about a specific resource in Azure. Although Postman can also be used for this purpose, here is an example of how to make these requests using PowerShell.
First, log in to your Azure account with the following command:
1Connect-AzAccount
Set the subscription context if you have multiple subscriptions:
1Set-AzContext -Subscription "<SubscriptionId>"
Get the current token:
1# Get the current token
2$Token = (Get-AzAccessToken).Token
Make the authorization header:
1# Set the authorization header
2$Headers = @{
3 Authorization = "Bearer $Token"
4}
Define wich resource you want to query. In this example, I want to get properties of my storage account, in a resource group in my subscription.
To get the API url and properties, I am using the REST API reference documentation: Azure REST API reference documentation | Microsoft Learn.
To construct the API URL I will substitute subscriptionId, resource group, and storage account with proper values.
1$Uri = "https://management.azure.com/subscriptions/{SubscriptionId}/resourceGroups/{ResourceGroupName}/providers/Microsoft.Storage/storageAccounts/{accountName}?api-version=2023-01-01"
Finally, use Invoke-WebRequest command for the API call:
1Invoke-WebRequest -Method GET -UseBasicParsing -Uri $Uri -Headers $Headers
JSON content of the request for the storage account properties can be accessed with:
1(Invoke-WebRequest -Method GET -Uri $Uri -Headers $Headers).Content
Here is a full code:
1Connect-AzAccount
2
3Set-AzContext -Subscription "<SubscriptionId>"
4
5# Get the current token
6$Token = (Get-AzAccessToken).Token
7
8# Set the authorization header
9$Headers = @{
10 Authorization = "Bearer $Token"
11}
12
13$Uri = "https://management.azure.com/subscriptions/{SubscriptionId}/resourceGroups/{ResourceGroupName}/providers/Microsoft.Storage/storageAccounts/{accountName}?api-version=2023-01-01"
14
15$Result = (Invoke-WebRequest -Method GET -Uri $Uri -Headers $Headers)
16
17If ($Result.StatusCode -eq "200"){
18 $Result.Content
19}